Comprehensive Debugging Guide: Mastering Error Resolution Like a Pro
When working in programming, you may encounter errors—or more colloquially, bugs. This is entirely normal in the world of programming. However, the real challenge begins at this point: as a programmer, do you have the skills to resolve these errors effectively?
In the debugging process, specific tools and techniques are used to identify and resolve errors.
What Is Debugging, and Why Is It Important?
As mentioned earlier, debugging refers to the process of identifying and fixing errors in the source code of a program. These errors can arise from typos, logical mistakes, or syntax issues in the code. Mastering debugging skills helps programmers reach solutions faster and prevents runtime problems
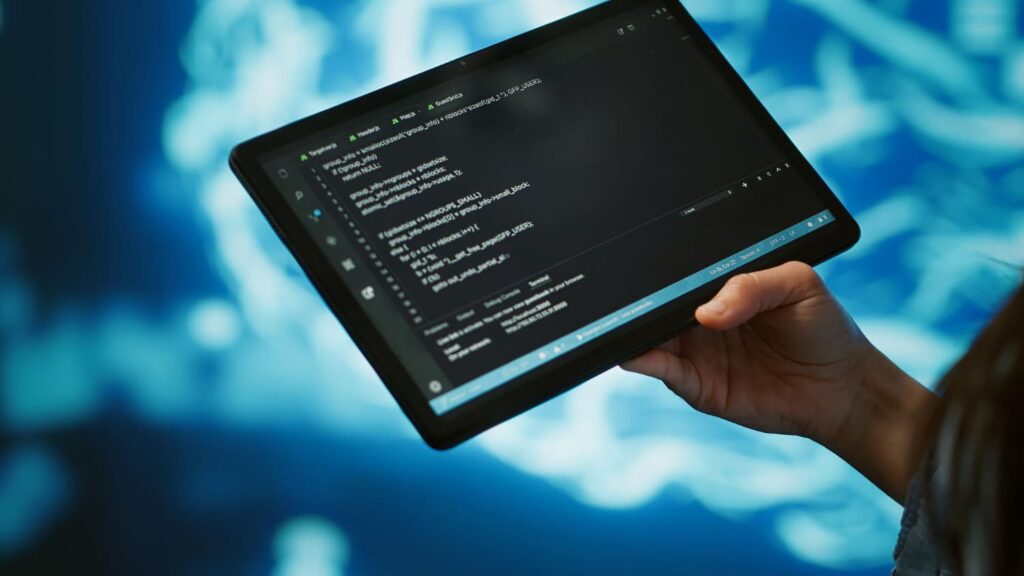
Benefits of Professional Debugging
You may wonder, What are the advantages of this skill? Let’s explore this question further:
- Reduced Development Time: Effective debugging shortens the time required to identify and fix errors.
- Improved Code Quality: Debugged and error-free code enhances program performance and reduces runtime issues.
- Deeper Learning: Debugging helps you better understand the principles and logic of programming languages.
Types of Errors in Programming
To fix errors, it’s essential to first familiarize yourself with their types:
- Syntax Errors: These errors occur when the code violates the grammar rules of the programming language.
- Logical Errors: These arise from mistakes in the program’s logic, causing incorrect behavior.
- Runtime Errors: These happen during program execution and may lead to crashes or unexpected stops.
- Semantic Errors: These occur when the code is syntactically correct but produces incorrect results due to meaning-related issues.
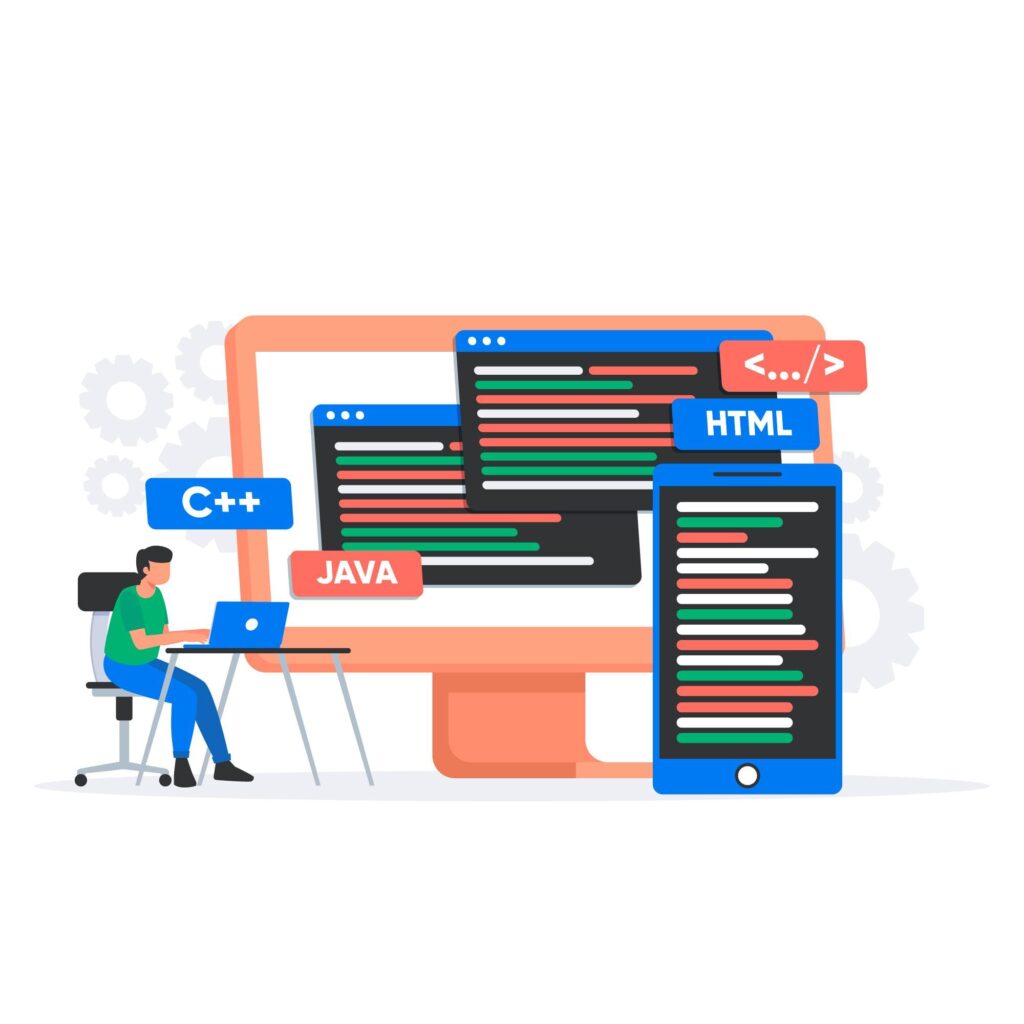
Debugging Tools
After identifying the type of error, the next step is to choose the best tool to resolve the bug. Below are some popular debugging tools:
Built-in Tools in Integrated Development Environments (IDEs)
IDEs like Visual Studio Code, PyCharm, and Eclipse come with built-in debugging tools, offering features like Breakpoints, Step Into, Step Over, and Watch Variables.
Standalone Debugger Tools
Tools such as GDB (for C and C++) and WinDbg (for Windows) allow you to debug code independently of specific IDEs.
Browser Consoles
For web developers, browser consoles like Google Chrome DevTools provide a powerful platform to identify and resolve errors in JavaScript, HTML, and CSS.
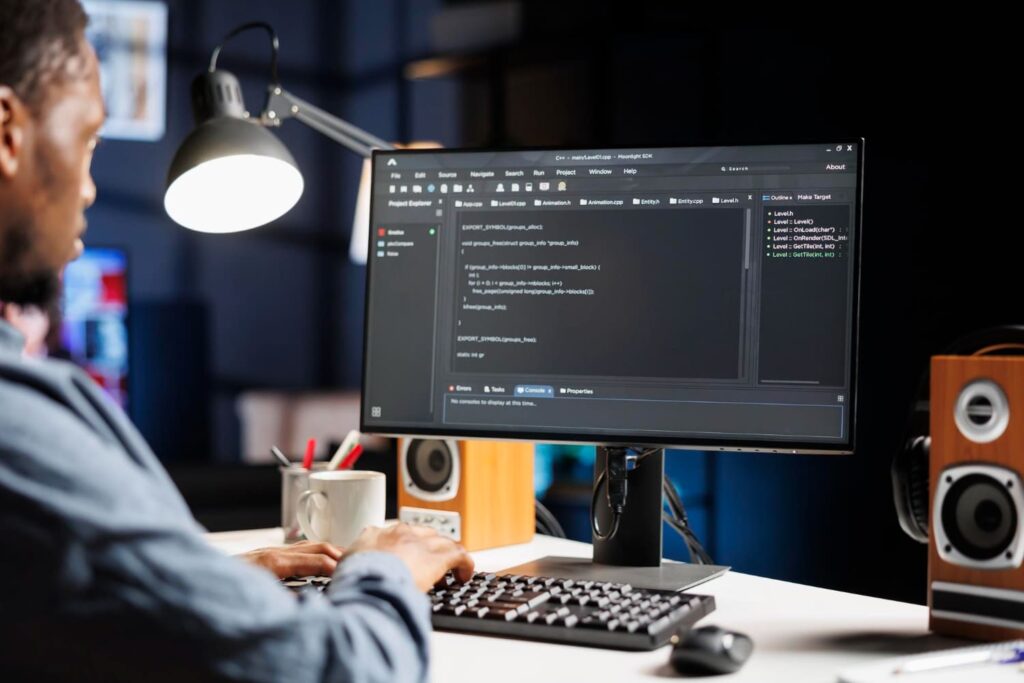
Debugging Step by Step
Now that we’ve covered the fundamentals, it’s time to walk through a debugging process step by step.
1. Review the Code:
The first step in debugging is carefully reviewing the code. Check whether your code is syntactically correct and ensure all functions and variables are being used properly.
2. Use Breakpoints:
Breakpoints are locations in your code where the program pauses execution. By using these, you can examine your code step by step, checking variable values and outputs at each stage.
3. Leverage error messages:
When the program runs, error messages appear. Carefully read these messages to determine the cause of the error. Programming languages like Python provide detailed error messages that are incredibly helpful for identifying issues.
4. Analyze variables and values:
During program execution, monitor the state of variables and their values. Debugging tools allow you to track each variable’s value at different stages and verify if it aligns with expected outcomes.
5. Logging Techniques:
In some cases, using logging techniques to display variable values and outputs during program execution can be beneficial. This approach makes it easier to identify errors.
6. Divide and Conquer Technique:
When faced with a complex error, break your code into smaller sections and test each individually. This method helps you quickly isolate the root cause of the issue.
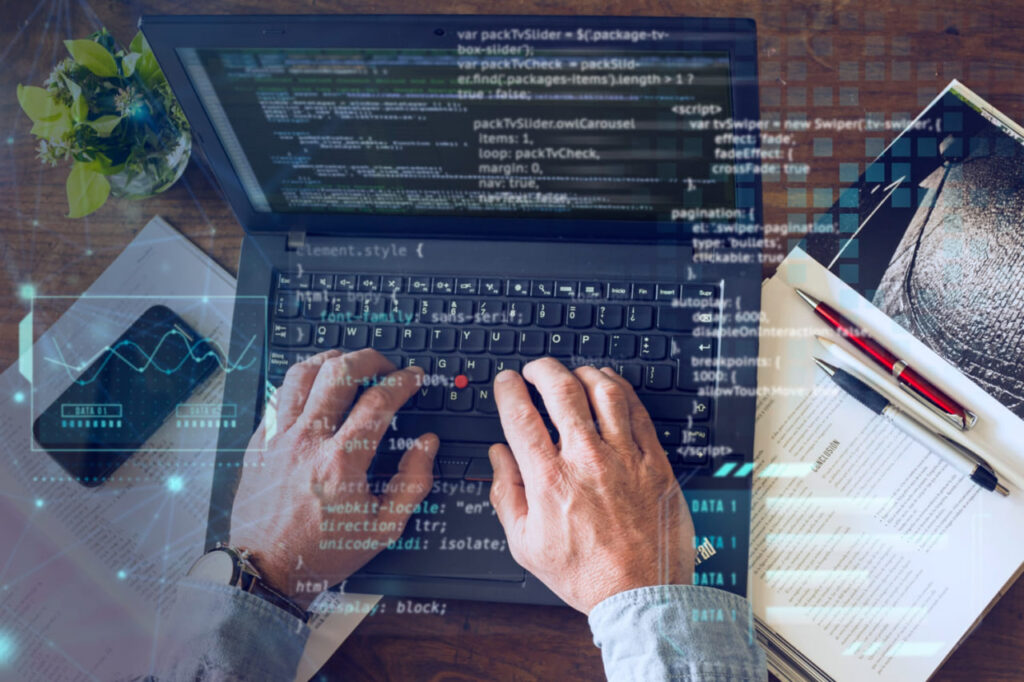
Tips and Tricks for Professional Debugging
1. Timing Tests and Debugging
In the software development process, treat debugging as part of the programming cycle. Debug your code after every significant change to ensure stability.
2. Use Monitoring Tools
In large-scale projects, employ monitoring and logging tools to identify issues in real time.
3. Document Changes
Document every small change to your code so that, in case of issues, you can easily trace the cause.
Debugging is an essential skill for every programmer, significantly improving code quality and reducing development time. By mastering debugging tools and techniques, you can fix errors like a pro and deliver higher-quality projects.
We also invite you to read our article on tools for learning and improving your English language skills!